Data Science Training in Noida
Appwars Technologies provides expert-led training in Noida to help you master data science. Certification, placement assistance, & practical projects are all covered.
Certification Partners

Our Students Placed In These Companies
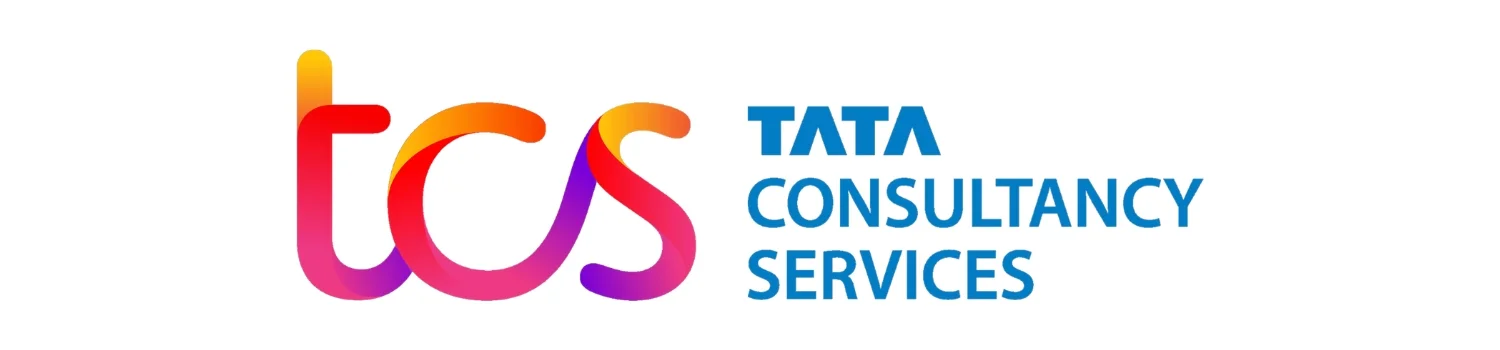
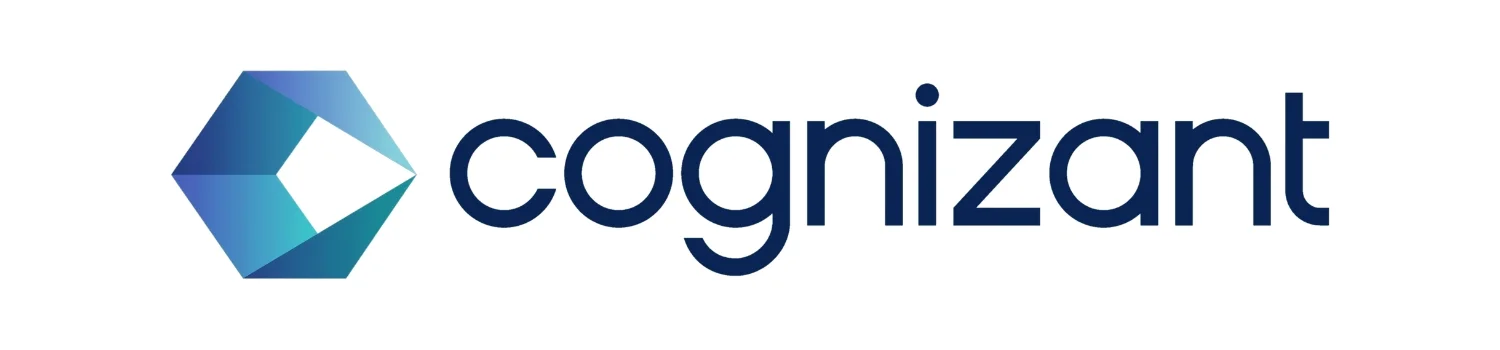
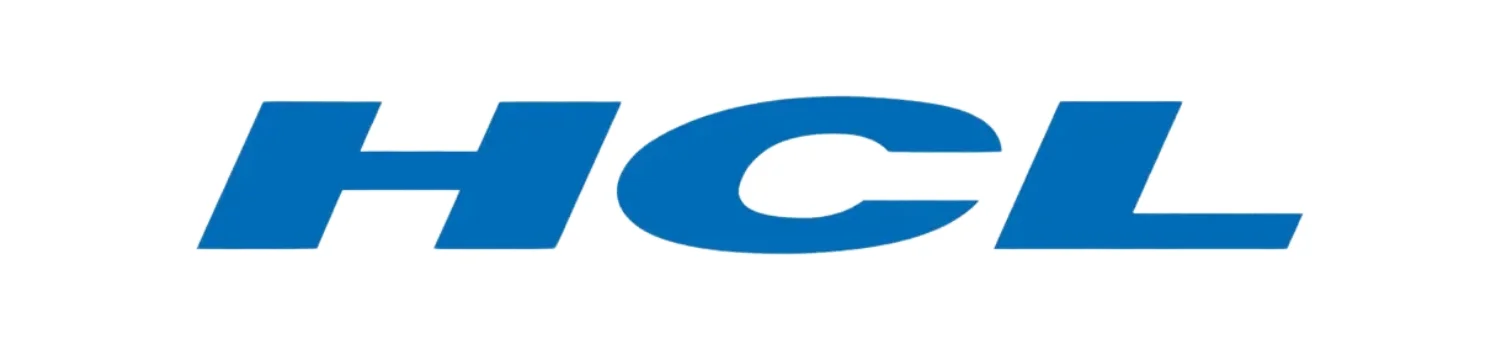
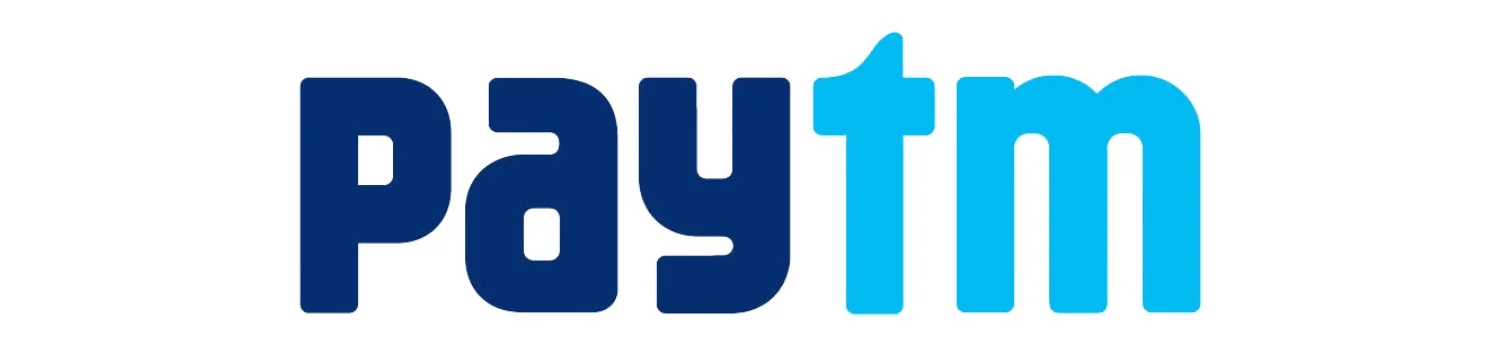
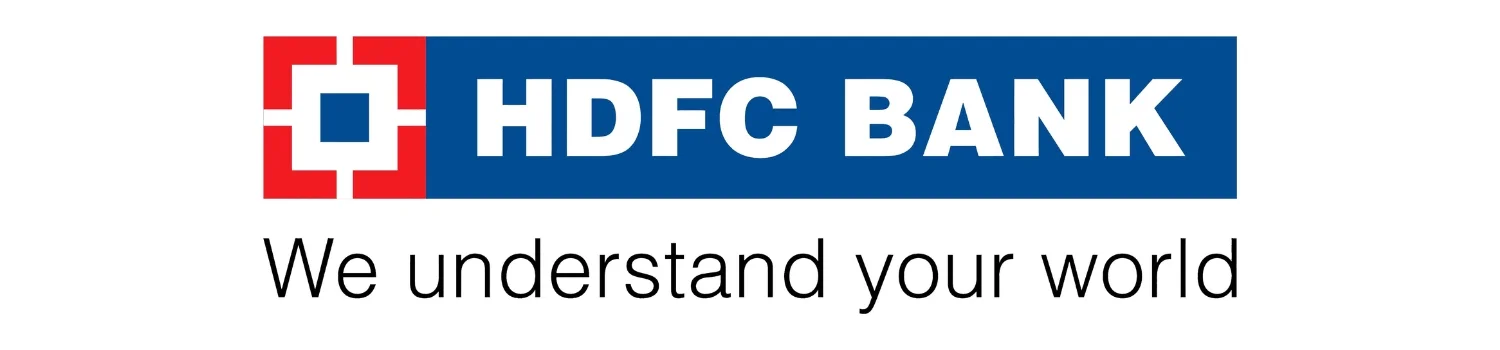
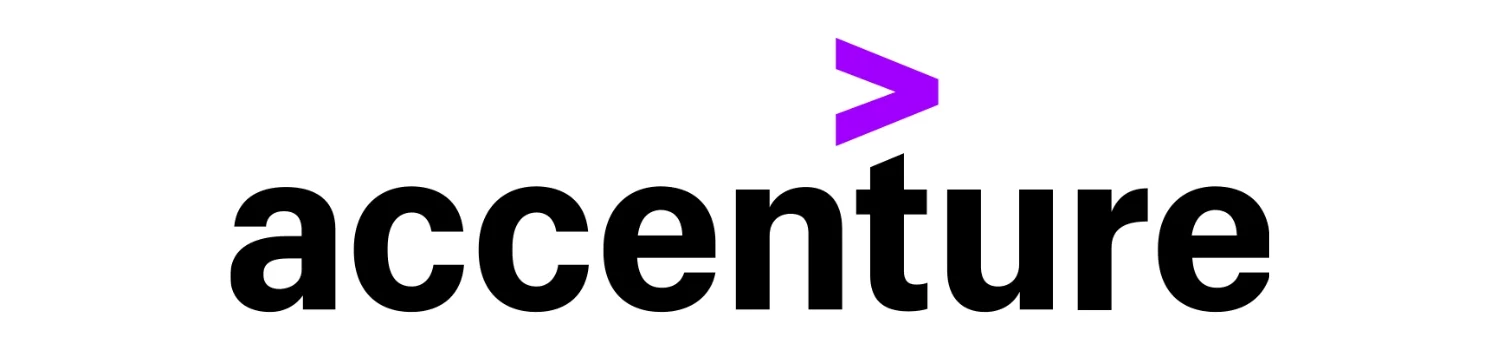
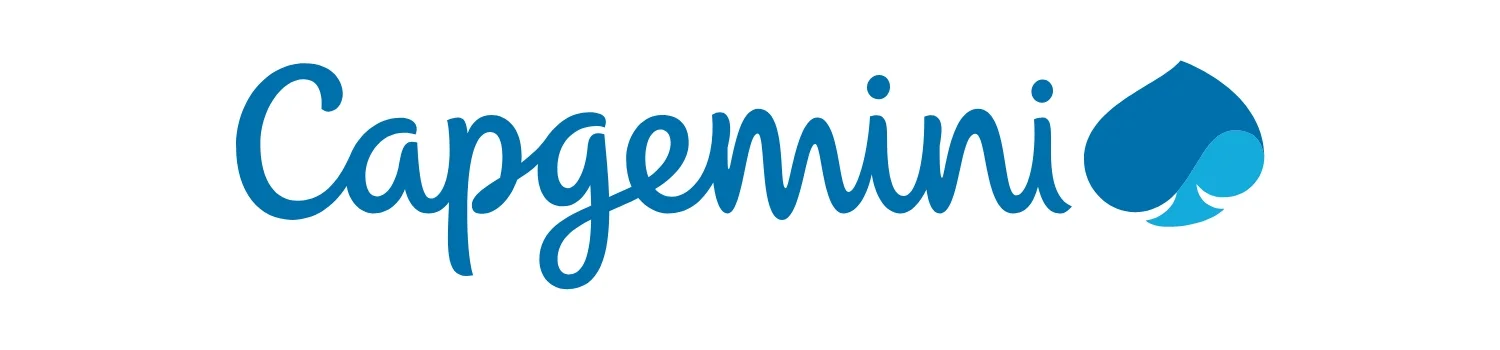
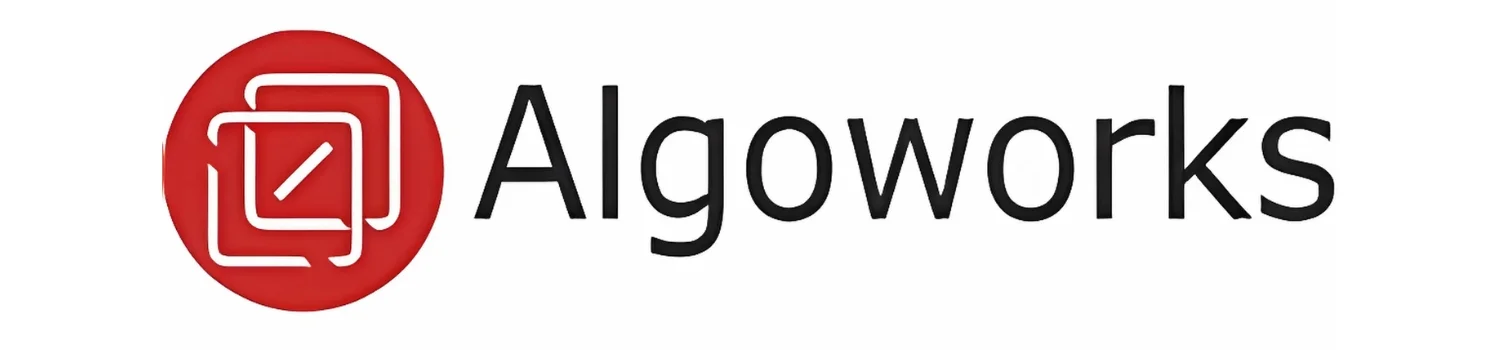
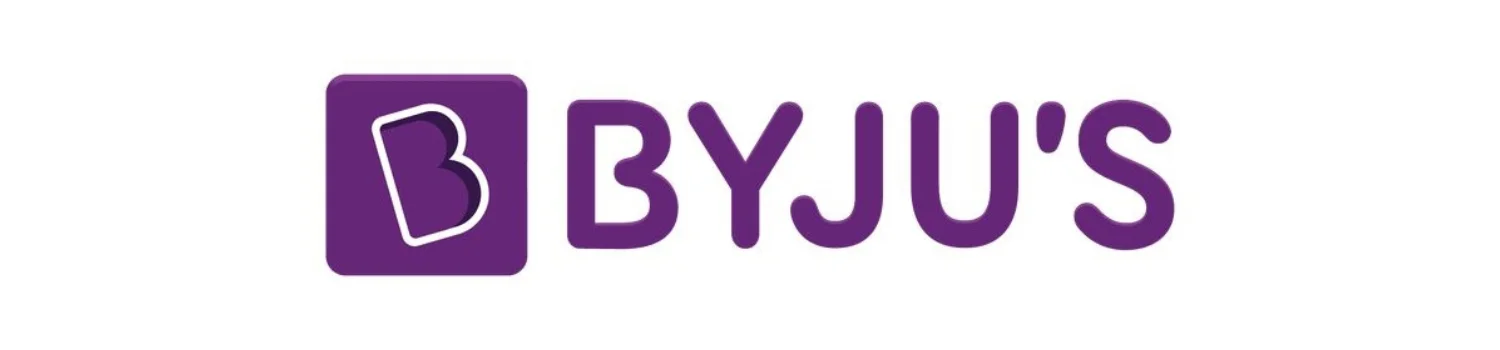
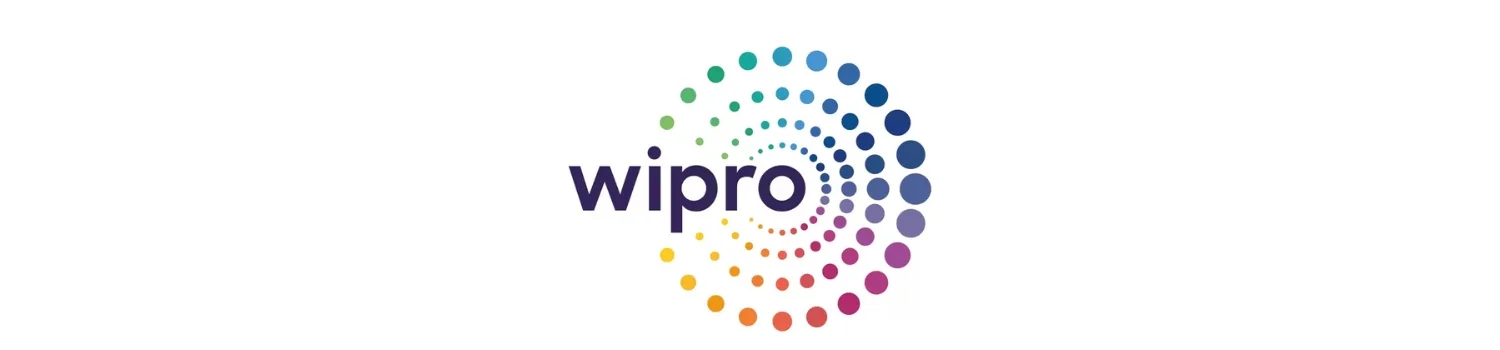
Data Science Course Content
- Python basics: syntax, data types, operators, control structures, functions
- Object-oriented programming (OOP)
- File handling, exception handling
- Modules, regular expressions
- Lists, Tuples, Sets, Dictionaries
- Built-in methods and list comprehensions
- Creating databases & tables, CRUD operations
- Joins, constraints, queries, and keys
- NumPy: Arrays, slicing, indexing, reshaping
- Pandas: Series, DataFrames, data cleaning, filtering, plotting
- Matplotlib & Seaborn: Data visualization, plots, grids
- Distributions (Normal, Poisson), Hypothesis testing
- T-test, ANOVA, Chi-Square, Central Limit Theorem
- Supervised: Linear & Logistic Regression, Decision Trees, KNN, SVM, Naive Bayes
- Unsupervised: K-Means, Hierarchical, DBSCAN, PCA
- Model evaluation: Accuracy, Precision, Recall, F1-score
- Ensemble models: Random Forest, AdaBoost, Gradient Boosting, XGBoost
- Artificial Neural Networks (ANN)
- Training algorithms: SGD, Adam, RMSprop
- CNN: Image classification, detection
- RNN/LSTM/GRU: Sequential data handling, NLP applications
- Tokenization, stemming, BoW, TF-IDF
- Word embeddings: Word2Vec, GloVe
- Transformers & BERT
- NLP tools: NLTK, SpaCy, TensorFlow
- Autoencoders (Vanilla, Sparse, Variational)
- GANs (Generative Adversarial Networks)
- Image processing, Object detection (YOLO, SSD), Image segmentation
- OpenCV, CNN implementation for real-time object detection
- Overview of Generative AI & how it works
- Introduction to LLMs: GPT, BERT, LLaMA, Claude
- Applications in chatbots, content creation, AI art
Our Placed Students

Skill & Tools That You will Learn
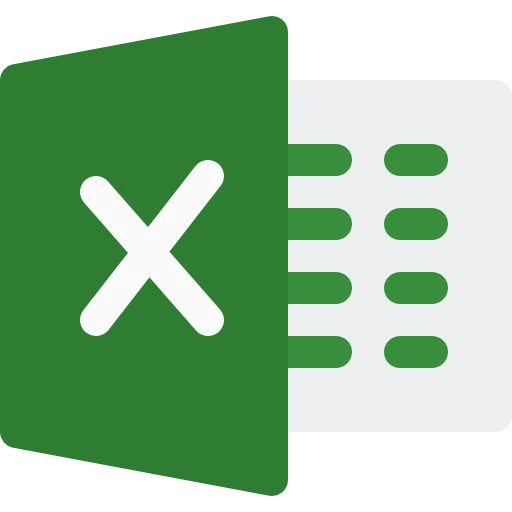
Excel
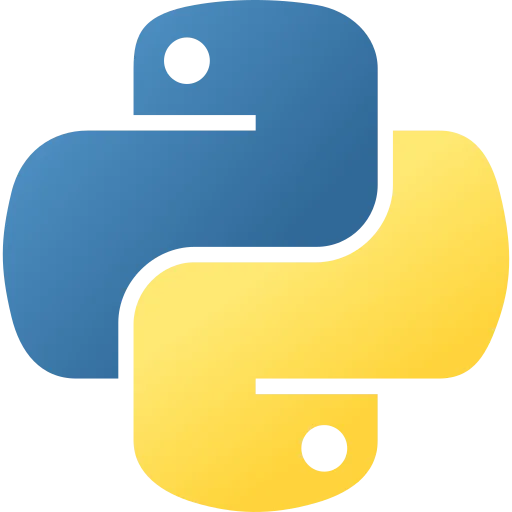
python

Power Bi
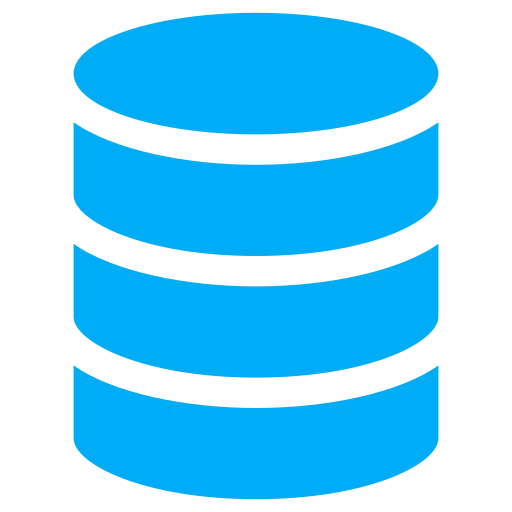
SQL

Tableau
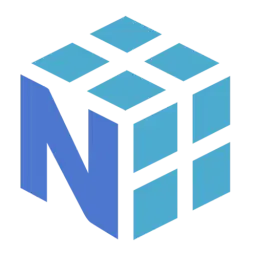
Numpy
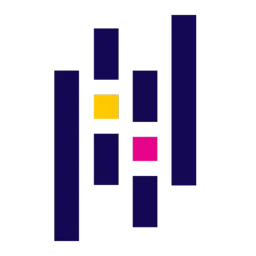
Pandas
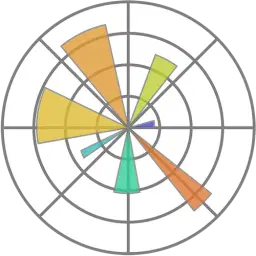
Matplotlib
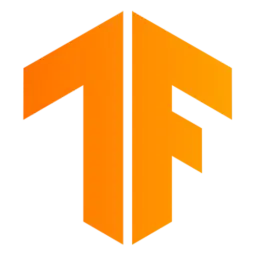
TensorFlow

Git Hub
Why Choose us?
Our Process

Introduction to Data Science
Data science is an interdisciplinary field that combines statistics, computer science, and domain expertise to extract meaningful insights from vast amounts of data. In today’s digital world, organizations are inundated with data generated from various sources, including social media, transactions, and IoT devices. This burgeoning data landscape amplifies the need for skilled professionals who can analyze and interpret complex datasets. The significance of data science cannot be overstated; it is instrumental in driving decision-making processes, predicting trends, and enhancing operational efficiencies across multiple sectors.
The applications of data science are extensive and diverse, permeating industries such as finance, healthcare, retail, and technology. In finance, for instance, data scientists analyze consumer behavior to identify credit risks and develop more robust fraud detection systems. In healthcare, predictive analytics is utilized to foresee outbreaks and improve patient care through personalized treatment plans. Similarly, in retail, data science plays a pivotal role in inventory management and customer segmentation, helping businesses optimize their strategies. The versatility of data science illustrates its transformative potential, which underscores the importance of acquiring comprehensive training in the field.
As demand for data science expertise continues to escalate, pursuing a data science course in Noida is an excellent step for aspiring data scientists. Such training often entails a deep dive into programming languages, statistical analysis, machine learning algorithms, and data visualization techniques. By establishing a solid foundation in these areas through effective data science training in Noida, individuals can equip themselves with the knowledge essential for navigating the complex data landscapes of the future.
Why Choose Appwars Technologies for Data Science Training?
Appwars Technologies stands out as a premier choice for data science training in Noida, offering a comprehensive program that equips learners with the necessary skills to excel in the ever-evolving field of data science. One of the most significant advantages of choosing Appwars is its team of highly experienced instructors, who bring a wealth of knowledge to the training sessions. With backgrounds in data science, machine learning, and artificial intelligence, these professionals not only impart theoretical knowledge but also share practical insights drawn from their industry experiences.
The training methodology adopted by Appwars Technologies is distinctly practical. The emphasis on hands-on learning ensures that students engage with real-world datasets and tools used in contemporary data science practices. This practical approach is further enhanced by industry-relevant projects, which form a core component of the data science course in Noida. By participating in projects that tackle authentic business challenges, learners gain valuable experience and confidence to manage data-driven tasks in their future careers.
Moreover, Appwars Technologies fosters a supportive learning environment that encourages interaction and collaboration among students. The institute provides ample opportunities for one-on-one mentorship, allowing learners to seek guidance whenever they encounter difficulties. This nurturing atmosphere not only facilitates better understanding of complex concepts but also helps build a strong network among aspiring data scientists.
In summary, the combination of experienced instructors, a hands-on practical approach, engaging industry-relevant projects, and a supportive learning atmosphere makes Appwars Technologies an ideal choice for individuals looking to pursue data science training in Noida. This unique blend of features prepares students to successfully navigate the intricacies of the data science domain and advance their careers effectively.
Data Science Course Curriculum Overview
The data science training in Noida offered by Appwars Technologies is meticulously designed to equip students with essential skills and knowledge required for a successful career in this rapidly evolving field. The course curriculum encompasses several key modules that cover both foundational and advanced topics.
Firstly, the program begins with an introduction to statistics, a crucial component of data science. Students will explore fundamental concepts including probability, distributions, hypothesis testing, and regression analysis. This module lays the groundwork for understanding data patterns and making informed decisions based on data interpretation.
Following the statistical module, the focus shifts to programming languages integral to data science. Students will receive in-depth training in Python and R, two of the most widely used languages in the industry. Practical exercises and projects will ensure that participants are proficient in using these languages for data manipulation, analysis, and implementation of algorithms.
The curriculum further includes an extensive module on machine learning, where students learn supervised and unsupervised learning techniques. Topics such as regression models, classification, clustering, and neural networks will be addressed, emphasizing the application of these techniques in solving real-world problems.
Additionally, data visualization plays a significant role in data interpretation. The course encompasses tools and techniques for creating informative visual representations of data using software such as Tableau and Matplotlib. This module equips students with the ability to communicate insights effectively.
Lastly, the program covers big data technologies, introducing students to frameworks like Hadoop and Spark. Understanding how to process and analyze large data sets is crucial in today’s digital landscape, making this knowledge imperative for aspiring data scientists.
Through this comprehensive curriculum, students of the data science course in Noida at Appwars Technologies will gain a robust understanding and practical experience, empowering them to excel in the field of data science.
Practical Training and Projects
In the field of data science, theoretical knowledge alone is insufficient for achieving proficiency; practical experience is equally essential. The data science training in Noida offered by Appwars Technologies places significant emphasis on hands-on projects and real-world applications. This approach ensures that students can effectively apply the concepts learned in the classroom to solve practical problems.
The training program includes a range of real-world projects designed to mimic the challenges faced by data professionals today. These projects often involve data collection, preprocessing, analysis, and the application of machine learning algorithms to derive meaningful insights. By working on these projects, students gain exposure to the entire data science workflow, enhancing their understanding of tools and techniques commonly used in the industry.
Moreover, the curriculum includes case studies that highlight the application of data science in various sectors such as healthcare, finance, and e-commerce. These case studies not only provide context to the theoretical knowledge but also help students appreciate the diverse ways in which data science impacts different industries. This exposure prepares them for potential career paths and equips them with the ability to tackle industry-specific problems.
Additionally, the lab sessions supplement these projects, allowing students to work on exercises that reinforce their skills in a controlled environment. During these sessions, trainers guide students in using advanced software and programming languages frequently utilized in data Science, ensuring they are well-prepared for real-world employment.
By integrating practical training with theoretical instruction, the data science course in Noida at Appwars Technologies assures that students emerge with a robust skill set and hands-on experience vital for a successful career in data science.
Expert Instructors and Industry Insights
One of the key components of a successful data science training in Noida is the caliber of the instructors leading the programs. At Appwars Technologies, the instructors are not just teachers but industry practitioners with substantial expertise in their fields. They come equipped with extensive academic qualifications, including advanced degrees in data science, statistics, computer science, and related domains. Their real-world experience enables them to provide valuable insights that traditional educational programs may overlook.
The instructors engage in ongoing professional development to stay abreast of the latest tools, techniques, and industry standards used in data science. This commitment to continuous learning allows them to impart knowledge that is relevant and immediately applicable to modern data challenges. During the data science course in Noida, these experts integrate case studies and practical exercises from their own professional experiences, bridging the gap between theory and practice.
Moreover, instructors at Appwars Technologies foster an interactive learning environment. They encourage students to ask questions, share ideas, and engage in discussions about current trends in data science. This collaborative approach not only enhances understanding but also prepares students to adapt to the evolving demands of the industry. As businesses increasingly rely on data-driven decision-making, instructors carefully align their teachings with market needs, focusing on skills that are highly sought after in today’s job market.
The workshops and seminars offered as part of the curriculum are an invaluable resource. They provide participants with firsthand exposure to complex data science projects, under the guidance of seasoned professionals. Students leave with a comprehensive understanding of both theoretical concepts and their practical applications, making them well-rounded candidates for a range of roles in the data science field.
Career Opportunities After Training
In today’s data-driven world, the demand for professionals skilled in data science continues to rise. Graduates of data science training in Noida can explore a myriad of career paths, each offering unique challenges and rewards. Among the most sought-after roles are data analyst, data scientist, machine learning engineer, and business intelligence analyst.
A data analyst plays a crucial role in interpreting complex datasets to derive actionable insights for organizations. They employ statistical techniques to analyze trends and provide recommendations. In Noida, data analysts can expect to earn an average salary ranging from ₹4 to ₹8 lakhs per annum, depending on their experience and expertise.
For those aspiring to become data scientists, the data science course in Noida equips individuals with advanced analytical skills and knowledge of machine learning algorithms. Data scientists are responsible for building predictive models and crafting data-driven strategies. The expected salary for this role can vary significantly, from ₹6 to ₹15 lakhs per annum, contingent upon one’s skills and years of experience.
Another valuable career option is that of a machine learning engineer. These professionals develop algorithms that allow computers to learn from data, automating the decision-making process. As machine learning becomes increasingly integral to various industries, demand for this role is expected to grow. Salaries for machine learning engineers in Noida can range from ₹8 to ₹16 lakhs per annum.
Lastly, business intelligence analysts are essential for translating data into strategic business decisions. By utilizing BI tools and data visualization techniques, they help organizations understand market trends and improve performance. Their salaries typically fall between ₹5 to ₹12 lakhs per annum.
The job market for data science professionals continues to flourish, with companies across various sectors seeking qualified candidates. With the right training, graduates can confidently embark on a rewarding career in this dynamic field, maximizing their earning potential and impact within their organizations.
Enrollment Process and Course Fees
The enrollment process for the data science training in Noida at Appwars Technologies is designed to be both straightforward and comprehensive, ensuring that prospective students are informed and well-prepared to embark on their educational journey. To begin the enrollment process, aspiring candidates are encouraged to visit the Appwars Technologies website where they can find detailed information on the data science course in Noida. Upon locating the course of interest, candidates must complete a registration form, providing necessary personal information and academic background.
After submission, candidates may be required to attend a counseling session, which can be conducted either in-person or online. This session is geared towards assessing the candidate’s suitability for the program, discussing their career aspirations in data science, and clarifying any doubts regarding the course content. Prerequisites typically include a background in mathematics, statistics, or information technology, although individuals from diverse academic fields are often welcome, provided they demonstrate a keen interest in data science.
As for course fees, Appwars Technologies offers competitive pricing that reflects the quality of training provided. The standard fee structure for the data science training in Noida consists of a one-time payment covering all course materials, lectures, and access to practical sessions. Currently, the fees are set at an affordable rate with various payment options available, including credit/debit cards, bank transfers, and installment plans. There are also early bird discounts and referral bonuses that can significantly reduce the overall cost. This flexibility allows students to choose an option that best fits their financial capabilities, ensuring that high-quality education in data science remains accessible to all.
Who can apply for the course?
BE / BTech / MCA passed aspirants to make their careers as Web Developers / Data Scientists
IT-Professionals who want to get a career as a Programming Expert
Professionals from non-IT bkg, and want to establish in IT
Candidates who would like to restart their career after a gap
Web Designers for the next level of their career.

Get Certified to boost your Professional Growth
Got more questions?
Talk to our team directly
Contact us and our academic counsellor will get in touch with you shortly
Our Gallery
Student Testimonials
EXCELLENT Based on 490 reviews Krish Bhatia2025-07-04Trustindex verifies that the original source of the review is Google. I am krish bhatia from trinity institute of professional studies they give us the good information on machine learning Shourya Jain2025-07-04Trustindex verifies that the original source of the review is Google. I shourya jain from TIPS dwarka, cam here for an industrial visit, i was given a small lecture by Aman sir and it was quite enlightening Abhishek Kumar2025-07-04Trustindex verifies that the original source of the review is Google. Best experience Domain machine learning By Aman Sir Name-Abhishek Kumar College -Trinity institute of Professional studies Sandeep2025-07-04Trustindex verifies that the original source of the review is Google. Best experience Domain learning in Machine learning Name sandeep College-trinity institute of professional studies dwarka sec 9 Chahat Prasher2025-07-04Trustindex verifies that the original source of the review is Google. Chahat (Trinity Institute of Professional Studies) Training by Aman Domain - AI/Ml Khushi Mittal2025-07-02Trustindex verifies that the original source of the review is Google. Completed a 6-week Core Java course under Shahnawaz Sir — the concepts were taught with clarity, real-world examples, and strong focus on fundamentals. Grateful for the valuable learning experience! Shaily Pandey2025-07-01Trustindex verifies that the original source of the review is Google. Sachin Sir provided excellent counseling." His way of explaining things was very clear and easy to understand. He listened to my problems attentively and then gave very practical and inspiring advice. His words boosted my confidence, and the confusion I had about my career is now completely clear. I am truly satisfied and would highly recommend him to anyone who is confused about their future or career. Thank you, Sachin Sir! Sahili Hawaldar2025-06-30Trustindex verifies that the original source of the review is Google. i was never a fan of coding but since i started learning coding from aman sir i have started liking coding He is a very good teacher he makes concept easy to understand right now i am studying python from him and i will suggest to go for appwars for any technical course Vijay Solanki2025-06-28Trustindex verifies that the original source of the review is Google. Thankyou APPWARDS for providing the opportunity of placement for java developer Chandan Pandey2025-06-27Trustindex verifies that the original source of the review is Google. I have attend the test with Java languageVerified by TrustindexTrustindex verified badge is the Universal Symbol of Trust. Only the greatest companies can get the verified badge who has a review score above 4.5, based on customer reviews over the past 12 months. Read more